how to scrape linkedin data using python? LinkedIn, the world’s largest professional network, is an information treasure trove for recruiters, marketers, researchers, and salespeople. Whether to discover potential recruits for positions or to generate leads or understand market trends and competitor positions, the applications of LinkedIn data are many. But how can you access the information programmatically? That is where it starts with learning to scrape LinkedIn data using Python.
Python, as rich in libraries as it is, offers a robust and powerful way of scraping data from websites like LinkedIn. This tutorial will walk you through the fundamentals of scraping LinkedIn data using Python, from the tools and ethical issues needed to a step-by-step guide and best practices for avoiding blocks. Whether you must build a targeted list of professionals or need to reap industry insights, learning how to scrape LinkedIn data with Python can be a game-changer.
Table of Contents
Understanding LinkedIn’s Stance: Terms of Service and Ethical Scraping
Before delving into the nitty-gritty of scraping LinkedIn, it’s vital to tackle the elephant in the room: LinkedIn’s Terms of Service (ToS) and the ethics of data scraping. LinkedIn’s ToS clearly forbids scraping or duplicating profiles and data through whatever means available, including spiders or web crawlers. Breaking these terms can result in account limitations or lifetime bans.
Moreover, there are serious ethical consequences. LinkedIn information belongs to individuals and companies, and unauthorized access to it raises privacy concerns. There is a need to be careful about data privacy regulations like GDPR and CCPA, which govern how personal data can be collected and processed.
For a deeper dive into the legal aspects, we recommend reading our article: Is It Legal to Scrape LinkedIn?.
Key Takeaways for Ethical Scraping:
- Be Respectful of Privacy: Avoid scraping sensitive personal information or using scraped information for spam or unethical purposes.
- Be Courteous to LinkedIn’s ToS: Be aware of the risk, and this could be suspension of your account or prosecution.
- Avoid Flooding Their Servers: Avoid spamming their servers with an excessive number of requests within a short period. It is not only courteous but also keeps your IP from being banned.
- Prioritize Public Data: Scraping publicly available data that is not behind a login wall is less of an issue, though still against LinkedIn’s ToS. Most valuable LinkedIn data, however, does involve a login.
- Transparency: If you are scraping data for research, anonymize the data and be transparent about how you gathered the data.
Although this tutorial covers the technical method of scraping data on LinkedIn using Python, caution must be exercised, and one should be aware of the possible repercussions and prioritize ethical data handling. Firms typically prefer to deal with professional data providers or out-of-the-box solutions that navigate these problems with more security.
Tools and Libraries Required for LinkedIn Scraping in Python
To scrape LinkedIn information with Python, you will need some basic tools and libraries at your disposal. Here’s a quick run-down of the basics:
- Python: The programming language itself. Make sure you have Python 3.6 or later installed. Python’s readability and vast library set make it a great language for web scraping purposes.
- Requests: This is a light but robust HTTP library that allows you to send HTTP requests to LinkedIn’s servers in an effort to fetch web pages. It’s ideal for static content but won’t be sufficient on its own for LinkedIn, since it relies heavily on JavaScript.
- Beautiful Soup 4 (BS4): After you have the HTML content of a page (which you got with Requests or a browser automation tool), Beautiful Soup is the one that actually parses it for you. It constructs a parse tree out of an HTML or XML document and provides you with simple mechanisms to traverse, search, and manipulate the tree.
- Selenium: LinkedIn loads a lot of content dynamically using JavaScript. The Requests library on its own is incapable of executing JavaScript, so you won’t get the whole page content. Selenium is a web browser automation tool, so your script can invoke web pages in the way a real user would – clicking on buttons, filling forms, and scrolling. That implies it can execute JavaScript-loaded content. You’ll need a WebDriver for whatever browser you’re using (e.g., Google Chrome’s ChromeDriver or Mozilla Firefox’s GeckoDriver).
- Download WebDriver: ChromeDriver, GeckoDriver
- LXML: Although Beautiful Soup can also use Python’s built-in HTML parser, LXML is a more capable and faster XML and HTML parser. It’s often used in conjunction with Beautiful Soup.
- Web Browser: You will need a web browser like Google Chrome or Mozilla Firefox for Selenium to automate.
- IDE (Integrated Development Environment): A good IDE like VS Code, PyCharm, or Jupyter Notebook will make coding, debugging, and project management much easier.
Considering Pre-built Solutions:
Development and maintenance of a LinkedIn scraper are complex and time-consuming due to LinkedIn’s anti-scraping policies and give you public data of each pages. Commercial software like a Linkedin Profile Scraper or a professional Linkedin Company Scraper will be more effective and handy solutions for users who need data without coding and maintenance hassles. The software usually offers IP rotation, CAPTCHA solution, and periodic updates to deal with LinkedIn changes.
LinkedIn Profile Scraper - Profile Data
Discover everything you need to know about LinkedIn Profile Scraper , including its features, benefits, and the different options available to help you extract valuable professional data efficiently.
Preparing Your Python Playground: Environment Setup
Prior to writing code to scrape linkedin data using python, you require a different and well-structured Python environment. This prevents project dependency conflicts and keeps your global Python installation clean.
1. Install Python:
In case you don’t have Python, get the most recent version of Python from the official Python website and install Python. Make sure you click on “Add Python to PATH” during installation if you’re installing on Windows.
2. Create a Virtual Environment:
A virtual environment is a distinct area for your Python projects.
- Open terminal or command prompt and navigate to your project directory:
- Create a virtual environment (e.g., named venv):
#python -m venv venv
- Activate the virtual environment:
- On Windows:
#venv\Scripts\activate
- On macOS and Linux:
#source venv/bin/activate
You’ll know the virtual environment is active when you see (venv) at the beginning of your terminal prompt.
3. Install Necessary Libraries:
With your virtual environment active, install the Python libraries mentioned in the previous section using pip:
pip install requests beautifulsoup4 selenium lxml pandas
We’ve added pandas here as it’s incredibly useful for storing and manipulating scraped data later on.
4. Install a WebDriver:
Selenium requires a WebDriver to interface with the chosen browser.
- ChromeDriver (for Google Chrome):
- Check your Chrome browser version.
- Download the corresponding ChromeDriver from the official site.
- Extract the chromedriver.exe (or chromedriver for Linux/macOS) and place it in a directory that’s in your system’s PATH, or directly in your project folder for simplicity.
- GeckoDriver (for Mozilla Firefox):
- Download the latest GeckoDriver from its GitHub releases page.
- Extract and place geckodriver.exe (or geckodriver) in your system’s PATH or project folder.
Your environment is now ready! You can start developing your Python script to scrape linkedin data using python. Remember to always activate your virtual environment (source venv/bin/activate or .\venv\Scripts\activate) when working on this project.
Step-by-Step Guide to Scraping LinkedIn Profiles Using Python
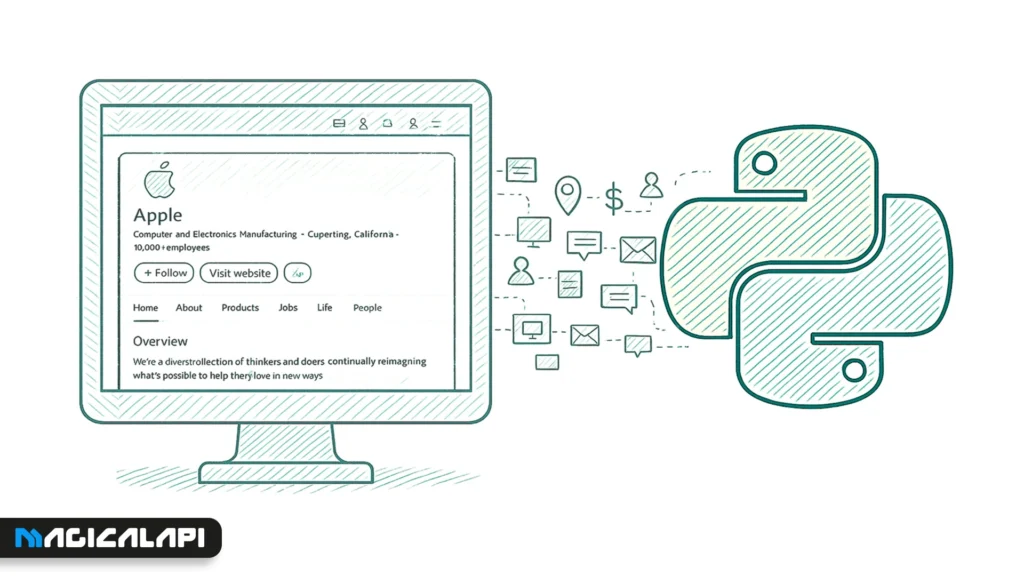
Now, let’s move to the essence of scraping LinkedIn data with Python. This part will give a conceptual step-by-step explanation. Keep in mind that scraping LinkedIn directly involves delicate management of login, dynamic content, and anti-bot protection.
Critical Note about Logging In:
Scraping LinkedIn successfully most of the time means being logged in because much of the useful data is inaccessible to non-logged-in accounts. It can be automated by logging in with Selenium but does risk your LinkedIn account.
- Manual Login First: One option is to manually login to LinkedIn in a browser session that will then be automated by Selenium.
- Automated Login (Use with Caution): You can automate the login by finding the email and password fields and the login button, and then using Selenium to fill them in and click. This does, however, make detection more probable.
Python
# Conceptual example of Selenium setup and login
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
import time
# Path to your WebDriver
webdriver_path = 'path/to/your/chromedriver' # or geckodriver
service = Service(executable_path=webdriver_path)
driver = webdriver.Chrome(service=service)
# Alternatively, if WebDriver is in PATH or same directory:
driver = webdriver.Chrome() # or webdriver.Firefox()
# --- Automated Login (Illustrative - HIGH RISK) ---
driver.get("https://www.linkedin.com/login")
time.sleep(2) # Allow page to load
username_field = driver.find_element(By.ID, "username")
username_field.send_keys("[email protected]")
password_field = driver.find_element(By.ID, "password")
password_field.send_keys("your_linkedin_password")
password_field.send_keys(Keys.RETURN) # Press Enter to submit
time.sleep(5) # Wait for login to complete and redirect
# It's better to use WebDriverWait for specific elements after login.
# Example: WebDriverWait(driver, 10).until(EC.presence_of_element_located((By.ID, "some_element_on_feed_page")))
# --- End of Automated Login ---
Always use placeholder or test accounts if experimenting with automated login, and be aware of the risks.
Step 1 of Scrape linkedin data using python: Searching for Profiles
Once logged in (manually or automated), you’ll typically start by searching for profiles.
- Navigate to the Search URL: You can construct a search URL directly or use Selenium to type into the search bar and apply filters.
- Using Selenium for Search:
# Conceptual: Assuming driver is initialized and logged in
driver.get("https://www.linkedin.com/feed/") # Go to feed or a relevant page
time.sleep(3)
search_bar = driver.find_element(By.XPATH, "//input[@aria-label='Search']") # XPATH can change
search_bar.send_keys("Software Engineer")
search_bar.send_keys(Keys.RETURN)
time.sleep(3) # Wait for search results
# You might need to click on "People" filter etc.
Step 2 of Scrape linkedin data using python: Extracting Profile URLs from Search Results
Search results pages will list multiple profiles. You need to extract the URLs of individual profiles to visit them.
- Inspect HTML: Use your browser’s developer tools (right-click > Inspect) to find the HTML structure containing the profile links. Look for <a> tags with href attributes pointing to /in/username.
- Parse with BeautifulSoup (after getting page source from Selenium):
# Conceptual:
page_source = driver.page_source
soup = BeautifulSoup(page_source, 'lxml')
profile_links = []
# This selector is highly likely to change and is just an example
for a_tag in soup.find_all('a', class_='app-aware-link', href=True):
href = a_tag['href']
if '/in/' in href and href not in profile_links and not href.startswith('/search'):
if not href.startswith('https://www.linkedin.com'):
href = 'https://www.linkedin.com' + href
profile_links.append(href)
print(profile_links)
- Handle Pagination: Search results span multiple pages. You’ll need to write logic to click the “Next” button and repeat the extraction process until no more pages are left.
Step 3 of Scrape linkedin data using python: Navigating to Individual Profile Pages and Scraping Data
Once you have a list of profile URLs, iterate through them, visit each page, and extract the desired information. This is a crucial part of how to scrape linkedin data using python.
For each profile URL:
driver.get(profile_url)
time.sleep(5) # Allow page to load fully. Use WebDriverWait for robustness.
profile_page_source = driver.page_source
profile_soup = BeautifulSoup(profile_page_source, 'lxml')
Common Data Points to scrape linkedin data using python (Selectors will vary and need updating):
- Name:
- Typically found in an <h1> tag near the top.
- Conceptual Selector: profile_soup.find(‘h1′, class_=’text-heading-xlarge’)
- Headline/Title:
- Usually a div or p tag below the name.
- Conceptual Selector: profile_soup.find(‘div’, class_=’text-body-medium break-words’)
- Location:
- Conceptual Selector: profile_soup.find(‘span’, class_=’text-body-small inline t-black–light break-words’)
- About Section:
- Often within a section identifiable by an ID like emberXXX or a specific class. You might need to click “See more” if the content is truncated. Selenium can handle this click.
- Conceptual Selector for the section: profile_soup.find(‘section’, id_starts_with=’ember’) then find relevant p tags.
- Experience:
- This is usually a list of roles. Each role will have a company name, title, dates, and description.
- You’ll need to find the main experience section (e.g., section with ID experience or similar).
- Then iterate through list items (li) representing each job.
- Conceptual Selectors within an experience item:
- Title: element.find(‘span’, class_=’mr1 t-bold’).find(‘span’, {‘aria-hidden’: ‘true’})
- Company: element.find(‘span’, class_=’t-14 t-normal’).find(‘span’, {‘aria-hidden’: ‘true’})
- Dates: element.find(‘span’, class_=’t-14 t-normal t-black–light’).find(‘span’, {‘aria-hidden’: ‘true’})
- Education:
- Similar structure to experience. Find the education section and iterate through entries.
- Skills:
- May require clicking a “Show all skills” button. Scrape the skill names.
These are important points to consider during scraping:
- Dynamic Content Loading & Scrolling: Some sections (like long experience lists or skills) might load more content as you scroll down. Use Selenium to scroll: driver.execute_script(“window.scrollTo(0, document.body.scrollHeight);”) and wait for new content to load.
- Pop-ups and Modals: Be prepared to handle unexpected pop-ups (e.g., “Connect,” “Message,” “Follow”) by instructing Selenium to click a close button or press the Escape key.
- Robust Selectors: LinkedIn’s HTML structure changes frequently. Avoid relying on highly specific or auto-generated CSS classes (like ember123). Prioritize IDs, stable class names, or structural relationships (e.g., “the div after the h2 with text ‘Experience'”). XPath can be very powerful for this.
Step 4 of Scrape linkedin data using python: Storing the Data (Covered more in “Exporting and Analyzing”)
As you scrape linkedin data using python, store the data in a structured format, like a list of dictionaries, where each dictionary represents a profile.
scraped_profiles = []
for profile_url in profile_links:
# ... navigate and scrape ...
profile_data = {
'name': name_text,
'headline': headline_text,
'location': location_text,
# ... other fields
'experience': [ {'title': '...', 'company': '...'}, ... ],
'education': [ {'institution': '...', 'degree': '...'}, ... ],
'skills': [skill1, skill2, ...]
}
scraped_profiles.append(profile_data)
time.sleep(random.uniform(5, 10)) # IMPORTANT: Add delays
This step-by-step guide offers a foundational understanding of how to scrape linkedin data using python. The key is patience, careful inspection of LinkedIn’s structure, and writing adaptable code.
Handling Anti-Bot Measures: Best Practices to Avoid Getting Blocked
LinkedIn employs sophisticated anti-bot measures to prevent automated scraping. If your scraper is too aggressive or easily identifiable as a bot, your IP address could be temporarily or permanently blocked, or your account could face restrictions. Here are best practices to minimize these risks when you scrape linkedin data using python:
- Mimic Human Behavior (Crucial!):
- Random Delays: Add random delays between requests and actions (e.g., time.sleep(random.uniform(3, 7))). Don’t hit pages too quickly. Humans don’t browse at lightning speed.
- Simulate Clicks, Scrolls, and Mouse Movements: Selenium allows for more human-like interactions than just fetching pages. If possible, simulate these.
- Vary Navigation Patterns: Don’t always follow the exact same path through the site for every profile.
- Rotate IP Addresses (Proxies):
- Making too many requests from a single IP address is a major red flag. Use a pool of proxy servers (residential proxies are often preferred for sites like LinkedIn as they look like real user IPs).
- There are many proxy providers. You can configure Selenium to use proxies.
# Conceptual proxy setup with Selenium
from selenium import webdriver
PROXY = "ip_address:port"
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument(f'--proxy-server={PROXY}')
driver = webdriver.Chrome(options=chrome_options)
- Rotate through your list of proxies for different requests or sessions.
- Use Realistic User-Agents:
- The User-Agent string in an HTTP request tells the server about your browser and OS. Rotate User-Agent strings to make your requests look like they’re coming from different browsers/devices.
- Maintain a list of common, up-to-date User-Agent strings.
# Conceptual user-agent setup with Selenium (Chrome)
from selenium import webdriver
user_agent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/98.0.4758.102 Safari/537.36"
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument(f'user-agent={user_agent}')
driver = webdriver.Chrome(options=chrome_options)
- Handle CAPTCHAs:
- LinkedIn might present CAPTCHAs if it suspects bot activity.
- Manual Intervention: The simplest (but not scalable) way is to solve them manually when they appear during development.
- CAPTCHA Solving Services: Integrate third-party services like 2Captcha or Anti-CAPTCHA. These services use human solvers or AI to solve CAPTCHAs for a fee. This adds complexity to your script.
- Detection Avoidance: The best strategy is to avoid triggering CAPTCHAs in the first place by being as human-like as possible.
- Work with Cookies and Sessions:
- Properly managing cookies can help maintain a logged-in session and make your activity appear more legitimate. Selenium handles cookies automatically within a browser session.
- You can also save cookies after a manual login and load them into Selenium for subsequent sessions, which can sometimes bypass the need to automate the login form itself repeatedly.
# Conceptual: Saving and loading cookies with Selenium
import pickle
# After manual login:
pickle.dump(driver.get_cookies(), open("linkedin_cookies.pkl", "wb"))
# In a new script/session:
driver.get("https://www.linkedin.com") # Go to a page on the domain first
cookies = pickle.load(open("linkedin_cookies.pkl", "rb"))
for cookie in cookies:
driver.add_cookie(cookie)
driver.refresh() # Refresh page to apply cookies
- Limit the Scale and Speed for scrape linkedin data using python:
- Don’t try to scrape thousands of profiles in a single day from one account/IP. Start small and scale gradually.
- Scrape during off-peak hours if possible, though LinkedIn traffic is global.
- The more data you try to extract per profile, and the faster you try to do it, the higher the risk.
- Use Headless Browsers Wisely:
- Headless browsers (browsers without a graphical user interface) are faster and consume fewer resources. Selenium supports headless mode.
- However, some websites, including LinkedIn, are better at detecting headless browsers. You might need to set additional options to make headless browsers look more like regular browsers (e.g., setting window size, user agent, and other navigator properties via JavaScript execution).
chrome_options.add_argument("--headless")
chrome_options.add_argument("--window-size=1920,1080") # Mimic a real display
chrome_options.add_argument("--disable-gpu") # Often used with headless
chrome_options.add_argument("--no-sandbox") # If running as root/admin
chrome_options.add_argument("--disable-dev-shm-usage") # Overcome limited resource problems
- Monitor and Adapt:
- LinkedIn frequently updates its website structure and anti-bot mechanisms. Your scraper will break eventually. Be prepared to regularly monitor its performance and update your selectors and logic.
- Implement logging in your script to track errors, blocked requests, or CAPTCHAs.
By implementing these best practices, you can significantly reduce the chances of being blocked while you scrape linkedin data using python. However, no method is foolproof, and the risk always remains.
LinkedIn Company Scraper - Company Data
Discover everything you need to know about LinkedIn Company Scraper , including its features, benefits, and the various options available to streamline data extraction for your business needs.
Exporting and Analyzing LinkedIn Data for Recruitment and Market Research
Once you’ve successfully navigated the complexities of how to scrape LinkedIn data using Python and extracted the information you need, the next crucial steps are exporting this data into a usable format and then analyzing it to derive insights.
1. Exporting Scraped Data
Your Python script will likely collect data into lists of dictionaries or custom objects. Here are common formats for exporting this data:
- CSV (Comma-Separated Values):
- Ideal for tabular data and easily imported into spreadsheets (Excel, Google Sheets) or databases.
- Python’s built-in csv module or the pandas library can be used.
# Using pandas (recommended for ease)
import pandas as pd
# Assuming 'scraped_profiles' is your list of dictionaries
df = pd.DataFrame(scraped_profiles)
df.to_csv('linkedin_data.csv', index=False, encoding='utf-8')
print("Data exported to linkedin_data.csv")
- JSON (JavaScript Object Notation):
- Good for hierarchical or nested data (like lists of job experiences within a profile).
- Readable by humans and easily parsed by most programming languages.
- Python’s built-in json module.
import json
with open('linkedin_data.json', 'w', encoding='utf-8') as f:
json.dump(scraped_profiles, f, ensure_ascii=False, indent=4)
print("Data exported to linkedin_data.json")
- Excel (XLSX):
- Similar to CSV but can support multiple sheets, formatting, and charts directly.
- Requires pandas along with an engine like openpyxl (install with pip install openpyxl).
Using pandas
df = pd.DataFrame(scraped_profiles)
df.to_excel('linkedin_data.xlsx', index=False, engine='openpyxl')
print("Data exported to linkedin_data.xlsx")
- Database:
- For larger datasets or ongoing scraping efforts, storing data in a database (e.g., SQLite, PostgreSQL, MySQL) is more robust.
- Libraries like sqlite3 (built-in) or SQLAlchemy (ORM) can be used.
Choosing the Right Format:
- For simple lists and quick analysis: CSV or Excel.
- For complex, nested data or API integration: JSON.
- For large-scale, persistent storage and querying: Database.
2. Analyzing LinkedIn Data
The true value of scraping LinkedIn data lies in how you analyze and apply it. Here are a few common use cases:
- Recruitment:
- Candidate Sourcing: Filter profiles based on skills, experience, location, education, and current title to find potential candidates.
- Talent Pool Building: Create a database of professionals in specific niches.
- Competitive Analysis: See where talent is moving from or to within your industry.
- Diversity and Inclusion: Analyze representation across different demographics (though be mindful of ethical data collection and bias).
- Market Research:
- Trend Analysis: Identify growing industries, in-demand skills, or popular job titles. For example, analyze the frequency of certain keywords in job descriptions or profiles.
- Competitor Intelligence: Analyze the employee base of competitor companies – their size, growth rate, key roles, and talent distribution.
- Skill Gap Analysis: Understand which skills are prevalent in a particular industry or role and identify potential gaps in your own organization or the market.
- Industry Benchmarking: Compare your company’s employee structure or talent pool against industry averages.
- Sales and Lead Generation:
- Identify Key Decision-Makers: Find professionals in target companies with specific job titles.
- Personalized Outreach: Use profile information (like common connections, shared interests, or recent activity) to craft more relevant outreach messages (though avoid spamming).
Tools for Analysis scrape linkedin data using python:
- Python with Pandas, NumPy, and Matplotlib/Seaborn: For data manipulation, statistical analysis, and visualization.
- Spreadsheet Software (Excel, Google Sheets): For basic filtering, sorting, and creating charts.
- BI Tools (Tableau, Power BI): For more advanced visualization and interactive dashboards.
- Natural Language Processing (NLP) Libraries (NLTK, spaCy): For analyzing textual data like job descriptions or “About” sections to extract keywords or sentiment.
Ethical Reminder: When analyzing and using scraped LinkedIn data, always refer back to ethical guidelines and privacy regulations. Ensure data is used responsibly and for the intended purpose.
By effectively exporting and analyzing the data you scrape LinkedIn data using Python, you can unlock valuable insights to inform your business strategies, recruitment efforts, and market understanding.
Conclusion: Scrape linkedin data using python
Python to scrape LinkedIn data opens doors for beneficial information in recruitment, market intelligence, and lead generation. In this article, utilizing tools like Selenium and BeautifulSoup, profile data scraping, and LinkedIn anti-bot features avoidance were explained.
Though possible technically, scraping LinkedIn using Python must be performed with respect for their Terms of Service and ethical data treatment. Effective, ethical scraping involves technical competence and expertise in web technologies as well as ethical conduct. Always employ random waits, proxies, and real user agents to avoid detection.
Keep in mind that LinkedIn’s structure shifts, and therefore scrapers have to be revised from time to time. For a more mechanized option, use professional LinkedIn Profile Scraper or Company Scraper tools like Magical API.
FAQ: How to Scrape LinkedIn Data Using Python
1. Is it legal to scrape data on LinkedIn?
Scraping of data from LinkedIn is against their ToS. Scraping publicly available information per se is in a legally gray area which has been interpreted differently in courts of law (e.g., hiQ Labs v. LinkedIn), but breaking ToS can result in account suspension and other consequences from LinkedIn. One must understand the risks and ethics involved. For additional information, view our post on “Is It Legal to Scrape LinkedIn?”.
2. What are the most critical Python libraries that I must employ in order to scrape LinkedIn?
The principal Python libraries used to scrape LinkedIn are:
Selenium: For simulating web browser activities, running JavaScript-rendered content, and navigating pages.
Beautiful Soup 4 (BS4): For parsing HTML and XML data scraped out by Selenium.
Requests: While not as used on LinkedIn itself due to its dynamic content, it can prove useful for other support HTTP requests or simpler sites.
Pandas: Useful for easy storing, manipulating, and exporting of scraped data in CSV or Excel format.
3. How do I avoid getting my account or IP blocked by LinkedIn?
To avoid blocks:
Simulate Human Behavior: Incorporate random delays between actions, simulate scroll, and randomize navigation patterns.
Use Proxies: Switch IP addresses using a good proxy server (residential proxies are trendiest).
Rotate User-Agents: Request look like coming from various browsers/OS.
Scrape with Moderate Speed: Not scrape big data with high speed.
Process CAPTCHAs: Be prepared for them, but best if we don’t trigger them.
Login Gently: Automated login is risky; use alternatives like stored cookies when available or manual login for session Selenium handles.
4. What’s real data to scrape from LinkedIn profiles?
You can typically attempt scraping publicly visible information on a profile you have view access to, such as:
Name, Headline, Location
About section
Experience (job title, companies, dates, descriptions)
Education (schools, degrees, dates)
Skills
Recommendations (if visible)
Connections count (generally an estimate)
The individual points are from the user’s privacy settings and what’s visible on LinkedIn.
5. Am I able to scrape LinkedIn without logging in?
LinkedIn limits data available to non-logged-in users very much. A great deal of the profile information and searching abilities require you to be logged in. While you can scrape some very superficial public landing pages, for any form of useful data scraping as presented in a tutorial for scraping LinkedIn data with Python, a logged-in session typically will be necessary. This does increase the risk to the account being accessed.